IF statement in Perl
The IF command is said to be a selection structure because it is a command that allows a certain action to be selected or not. That is, with the use of IF, some things are executed and some not, all depending on conditional tests that we will do.if( test ){ # code in case the test # be true or 1 }It works like this, first type if, then we open parentheses and inside it must have something that is true (1) or false (empty), we usually put a conditional test statement, that is, a comparison, which we study in the last tutorial of our course. Perl
Then we open braces: {}
If the conditional test is true, everything within these keys will be executed.
If the test is false, the IF command is simply ignored, perl skips it as if it did not exist.
What happens here is decision making. Perl evaluates whether the value is true or false and decides to execute a certain block of code or not.
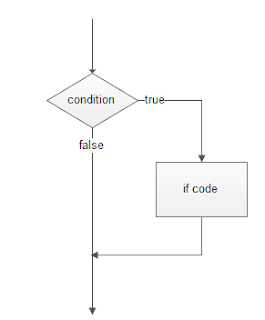
Let's see how this works in practice?
Example of using IF in Perl
You have been hired to create a script for a nightclub. In it, you ask the age of the person and if he is 21 or older, warns that he can enter.Let's store the person's age in the $age variable and ask for their age via <STDIN>.
Now we need to compare $ age with 18 to find out if it is greater than or equal to:
- $ age >= 21
Just put this in parentheses of IF.
Inside if, we only give a print warning that the person is bigger and can enter, see how our code was:
#!/usr/bin/perl print "Tye your age: "; chomp($age=<STDIN>); if($age>=18){ print "You can come in\n"; }
How to use IF in strings in Perl
You have to create a snippet of code where you will be prompted for a password for the user, and will allow them to enter the system only if they enter the correct password, which is rush2112.We will store the password in the $password variable and compare it with the string 'rush2112', if it is the same, enter the IF code and say it will enter the system, our code looks like this:
#!/usr/bin/perl print "Type your password: "; chomp($password=<STDIN>); if($password eq 'rush2112'){ print "Correct password, entering in the system...\n"; }
What if you enter the wrong password? It does not fall within the IF code, so it does not enter the system, simple as that.
Boolean Values in Perl
In fact, it does not have to be a conditional test, the important thing is to result in a boolean value:- True, true or 1
- False, false, empty or 0
The following IF will always be executed:
#!/usr/bin/perl if(1){ print "This IF always runs."; }The following IF, only programmers can see what's inside it, as it will never run:
#!/usr/bin/perl if(0){ print "Only Perl Programmers Can Read This."; }'Read the code': if true, execute the code below ...
Makes sense, doesn't it?
Note that now our scripts don't always work the same way as before ... they depend, they rely on a conditional test. We now have greater control over our little Perl programs.
No comments:
Post a Comment